How to Create Simple Calculator in Android Studio Java
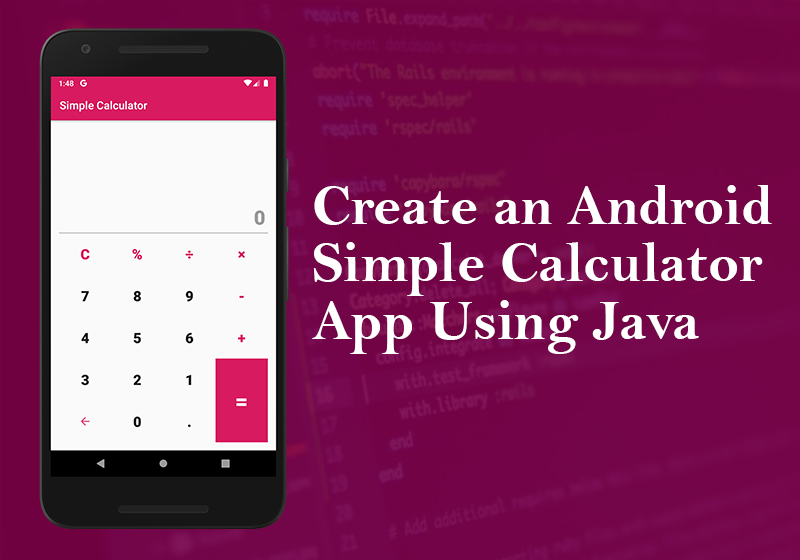
In this Tutorial we will learn how to create a simple calculator in android studio.Lets start
Step 1: Building the user interface
First we design our user interface using TextView, Button and ImageButton. TextView are used to display user input and result, Button and ImageButton are Used to get user Input.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="15dp"
android:weightSum="3"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:orientation="vertical"
android:layout_weight="1">
<TextView
android:id="@+id/display"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:padding="5dp"
android:gravity="end|bottom"
android:hint="0"
android:textSize="36dp"
android:fontFamily="sans-serif-black"
android:maxLines="4"
android:textColor="@android:color/black"
android:background="@android:color/transparent" />
<TextView
android:id="@+id/result"
android:layout_width="match_parent"
android:layout_height="0dp"
android:visibility="gone"
android:layout_weight=".5"
android:padding="5dp"
android:gravity="end|bottom"
android:text="="
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:maxLines="4"
android:textColor="@android:color/black"
android:background="@android:color/transparent" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="1px"
android:background="@android:color/black"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="2"
android:weightSum="5"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:orientation="horizontal"
android:weightSum="4">
<Button
android:id="@+id/button_clear"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="C"
android:textSize="26dp"
android:textColor="@color/colorAccent"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_bg"/>
<Button
android:id="@+id/button_modulas"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="%"
android:textSize="26dp"
android:textColor="@color/colorAccent"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_bg"/>
<Button
android:id="@+id/button_div"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="÷"
android:textSize="26dp"
android:textColor="@color/colorAccent"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_bg"/>
<Button
android:id="@+id/button_multi"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="×"
android:textSize="26dp"
android:textColor="@color/colorAccent"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_bg"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:orientation="horizontal"
android:weightSum="4">
<Button
android:id="@+id/button_7"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="7"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_8"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="8"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_9"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="9"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_sub"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="-"
android:textSize="26dp"
android:textColor="@color/colorAccent"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_bg"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:orientation="horizontal"
android:weightSum="4">
<Button
android:id="@+id/button_4"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="4"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_5"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="5"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_6"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="6"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_add"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="+"
android:textSize="26dp"
android:textColor="@color/colorAccent"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_bg"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="2"
android:orientation="horizontal"
android:weightSum="4">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_weight="3"
android:weightSum="2">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:orientation="horizontal"
android:weightSum="3">
<Button
android:id="@+id/button_3"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="3"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="2"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_1"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="1"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:orientation="horizontal"
android:weightSum="3">
<ImageButton
android:id="@+id/button_back"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:src="@drawable/back"
android:backgroundTint="@color/colorAccent"
android:background="@drawable/ripple_bg"/>
<Button
android:id="@+id/button_0"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="0"
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
<Button
android:id="@+id/button_dot"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="."
android:textSize="26dp"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_btn"/>
</LinearLayout>
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1">
<Button
android:id="@+id/button_equal"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="="
android:textSize="40dp"
android:textColor="@android:color/white"
android:fontFamily="sans-serif-black"
android:background="@drawable/ripple_equal_btn"/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
Step 2: Setup Resource file
1. Right click on drawable -> new -> Image Asset Choose Icon Type Action Bar and Tab Icons then click Click Art Image and select Which Icon Name is close then press ok and then name it back and change color and use this color code D81B60 finaly click next->Finish.
2. Right click on drawable -> new -> Drawable Resource File then name it ripple_bg and click Ok. You can see ripple_bg.xml file under your drawable folder. Copy the bellow code and paste it into your ripple_bg.xml.
<?xml version="1.0" encoding="utf-8"?>
<ripple xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:color="@color/colorPrimaryDark"
tools:targetApi="lollipop">
<item android:id="@android:id/mask">
<shape android:shape="rectangle">
<solid android:color="@color/colorPrimaryDark" />
<corners android:radius="0dp" />
</shape>
</item>
<item android:id="@android:id/background">
<shape android:shape="rectangle">
<gradient
android:angle="90"
android:endColor="@android:color/transparent"
android:startColor="@android:color/transparent"
android:type="linear" />
<corners android:radius="0dp" />
</shape>
</item>
</ripple>
3. Right click on drawable -> new -> Drawable Resource File then name it ripple_bg and click Ok. You can see ripple_btn.xml file under your drawable folder. Copy the bellow code and paste it into your ripple_btn.xml.
<?xml version="1.0" encoding="utf-8"?>
<ripple xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:color="#88000000"
tools:targetApi="lollipop">
<item android:id="@android:id/mask">
<shape android:shape="rectangle">
<solid android:color="#88000000" />
<corners android:radius="0dp" />
</shape>
</item>
<item android:id="@android:id/background">
<shape android:shape="rectangle">
<gradient
android:angle="90"
android:endColor="@android:color/transparent"
android:startColor="@android:color/transparent"
android:type="linear" />
<corners android:radius="0dp" />
</shape>
</item>
</ripple>
4. Right click on drawable -> new -> Drawable Resource File then name it ripple_bg and click Ok. You can see ripple_equal_btn.xml file under your drawable folder. Copy the bellow code and paste it into your ripple_equal_btn.xml.
<?xml version="1.0" encoding="utf-8"?>
<ripple xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:color="#000000"
tools:targetApi="lollipop">
<item android:id="@android:id/mask">
<shape android:shape="rectangle">
<solid android:color="#000000" />
<corners android:radius="0dp" />
</shape>
</item>
<item android:id="@android:id/background">
<shape android:shape="rectangle">
<gradient
android:angle="90"
android:endColor="@color/colorPrimaryDark"
android:startColor="@color/colorPrimaryDark"
android:type="linear" />
<corners android:radius="0dp" />
</shape>
</item>
</ripple>
Now your design look like this
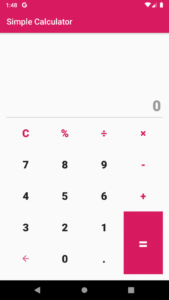
Step 3: Coding the Functionality
In your Java file, which is almost always MainActivity.java by default, head on over to the MainActivity extends AppCompatActivity class. Copy bellow code and paste into your MainActivity.java file. But without changing your project package name [ package com.andrious.simplecalculator; ]
import android.content.res.Configuration;
import android.graphics.Color;
import android.os.Bundle;
import android.util.TypedValue;
import android.view.View;
import android.widget.Button;
import android.widget.ImageButton;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
Double result_value = 0.0;
Boolean checker = true;
int orientation = 0;
TextView result, display;
Button button_0,button_1,button_2,button_3,button_4,button_5,button_6,button_7,button_8,button_9;
Button button_dot, button_clear, button_modulas, button_add, button_sub, button_multi, button_div, button_equal;
ImageButton button_back;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button_0 = findViewById(R.id.button_0);
button_1 = findViewById(R.id.button_1);
button_2 = findViewById(R.id.button_2);
button_3 = findViewById(R.id.button_3);
button_4 = findViewById(R.id.button_4);
button_5 = findViewById(R.id.button_5);
button_6 = findViewById(R.id.button_6);
button_7 = findViewById(R.id.button_7);
button_8 = findViewById(R.id.button_8);
button_9 = findViewById(R.id.button_9);
button_add = findViewById(R.id.button_add);
button_sub = findViewById(R.id.button_sub);
button_multi = findViewById(R.id.button_multi);
button_div = findViewById(R.id.button_div);
button_modulas = findViewById(R.id.button_modulas);
button_dot = findViewById(R.id.button_dot);
button_equal = findViewById(R.id.button_equal);
button_clear = findViewById(R.id.button_clear);
button_back = findViewById(R.id.button_back);
result = findViewById(R.id.result);
display = findViewById(R.id.display);
orientation = getResources().getConfiguration().orientation;
button_modulas.setEnabled(false);
entry_controller(checker);
button_0.setOnClickListener(new View.OnClickListener() {
public void onClick(View v){
display.setText(display.getText().toString() + "0");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_1.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "1");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_2.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "2");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_3.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "3");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_4.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "4");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_5.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "5");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_6.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "6");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_7.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "7");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_8.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "8");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_9.setOnClickListener(new View.OnClickListener() {
public void onClick(View v){
display.setText(display.getText().toString() + "9");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_dot.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + ".");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
button_add.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "+");
text_size_color_controller();
checker = true;
entry_controller(checker);
}
});
button_sub.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "-");
text_size_color_controller();
checker = true;
entry_controller(checker);
}
});
button_multi.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "*");
text_size_color_controller();
checker = true;
entry_controller(checker);
}
});
button_div.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText(display.getText().toString() + "/");
text_size_color_controller();
checker = true;
entry_controller(checker);
}
});
button_modulas.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
result.setText("=" + result_value / 100.0);
}
});
button_clear.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
display.setText("");
result.setText("");
result.setVisibility(View.GONE);
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP, 36F);
}
});
button_back.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String data = display.getText().toString();
if (data.length() > 0) {
display.setText(data.substring(0, data.length() - 1));
text_size_color_controller();
}
}
});
button_equal.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String data = display.getText().toString();
if (!data.endsWith("+") || !data.endsWith("-") || !data.endsWith("*") || !data.endsWith("/")) {
result_value = calculation(data);
result.setVisibility(View.VISIBLE);
result.setText("= " + String.format("%.5f", result_value).toString());
display.setTextColor(Color.parseColor("#bdbdbd"));
result.setTextColor(Color.parseColor("#000000"));
if (orientation == Configuration.ORIENTATION_LANDSCAPE) {
// In landscape
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP, 26F);
result.setTextSize(TypedValue.COMPLEX_UNIT_DIP, 20F);
} else {
// In portrait
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP, 26F);
result.setTextSize(TypedValue.COMPLEX_UNIT_DIP, 36F);
}
button_modulas.setEnabled(true);
}
}
});
}
public Void text_size_color_controller() {
display.setTextColor(Color.parseColor("#000000"));
result.setTextColor(Color.parseColor("#bdbdbd"));
if (orientation == Configuration.ORIENTATION_LANDSCAPE) {
// In landscape
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP,30F);
result.setTextSize(TypedValue.COMPLEX_UNIT_DIP,20F);
} else {
// In portrait
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP,36F);
result.setTextSize(TypedValue.COMPLEX_UNIT_DIP,26F);
}
button_modulas.setEnabled(false);
return null;
}
public Void entry_controller(Boolean b) {
if(b){
button_add.setEnabled(false);
button_sub.setEnabled(false);
button_multi.setEnabled(false);
button_div.setEnabled(false);
button_equal.setEnabled(false);
}else{
button_add.setEnabled(true);
button_sub.setEnabled(true);
button_multi.setEnabled(true);
button_div.setEnabled(true);
button_equal.setEnabled(true);
}
return null;
}
public static double calculation(final String str) {
return new Object() {
int pos = -1, ch;
void nextChar() {
ch = (++pos < str.length()) ? str.charAt(pos) : -1;
}
boolean eat(int charToEat) {
while (ch == ' ') nextChar();
if (ch == charToEat) {
nextChar();
return true;
}
return false;
}
double parse() {
nextChar();
double x = parseExpression();
if (pos < str.length()) throw new RuntimeException("Unexpected: " + (char)ch);
return x;
}
// Grammar:
// expression = term | expression `+` term | expression `-` term
// term = factor | term `*` factor | term `/` factor
// factor = `+` factor | `-` factor | `(` expression `)`
// | number | functionName factor | factor `^` factor
double parseExpression() {
double x = parseTerm();
for (;;) {
if (eat('+')) x += parseTerm(); // addition
else if (eat('-')) x -= parseTerm(); // subtraction
else return x;
}
}
double parseTerm() {
double x = parseFactor();
for (;;) {
if (eat('*')) x *= parseFactor(); // multiplication
else if (eat('/')) x /= parseFactor(); // division
else return x;
}
}
double parseFactor() {
if (eat('+')) return parseFactor(); // unary plus
if (eat('-')) return -parseFactor(); // unary minus
double x;
int startPos = this.pos;
if (eat('(')) { // parentheses
x = parseExpression();
eat(')');
} else if ((ch >= '0' && ch <= '9') || ch == '.') { // numbers
while ((ch >= '0' && ch <= '9') || ch == '.') nextChar();
x = Double.parseDouble(str.substring(startPos, this.pos));
} else if (ch >= 'a' && ch <= 'z') { // functions
while (ch >= 'a' && ch <= 'z') nextChar();
String func = str.substring(startPos, this.pos);
x = parseFactor();
if (func.equals("sqrt")) x = Math.sqrt(x);
else if (func.equals("sin")) x = Math.sin(Math.toRadians(x));
else if (func.equals("cos")) x = Math.cos(Math.toRadians(x));
else if (func.equals("tan")) x = Math.tan(Math.toRadians(x));
else throw new RuntimeException("Unknown function: " + func);
} else {
throw new RuntimeException("Unexpected: " + (char)ch);
}
if (eat('^')) x = Math.pow(x, parseFactor()); // exponentiation
return x;
}
}.parse();
}
}
Now every thing is done. Run and enjoy the calculator app.
Step 4: Function description
Fetching values from the elements into the working of our app
First declare necessary variable inside our class
Double result_value = 0.0;
Boolean checker = true;
int orientation = 0;
TextView result, display;
Button button_0,button_1,button_2,button_3,button_4,button_5,button_6,button_7,button_8,button_9;
Button button_dot, button_clear, button_modulas, button_add, button_sub, button_multi, button_div, button_equal;
ImageButton button_back;
Inside the onCreate function, we will fetch all values and assign them to our objects. We do this by the following code
button_0 = findViewById(R.id.button_0);
Here, we are fetching the value of ‘button_0’, which is the ID of our button zero, and storing it in the object ‘button_0’ that we created earlier. Though they are named the same, Android Studio can identify the correct one. But if it gets confusing for you, feel free to give either one of them any other names.
We do the same with our TextView.
result = findViewById(R.id.result);
Changing the TextView value by pressing the buttons.
button_0.setOnClickListener(new View.OnClickListener() {
public void onClick(View v){
display.setText(display.getText().toString() + "0");
text_size_color_controller();
checker = false;
entry_controller(checker);
}
});
We use OnClickListener for this part of the program. This is what happens when you push zero. The TextView, first already fetches the value that it is already displaying, null in this case, and then adds 0 to it.
Text Font size control when change the phone orientation.
In onCreate method we initialize it and get the device current orientation.
orientation = getResources().getConfiguration().orientation;
Finally use conditional statement in button_equal onClick function based on device orientation.
if (orientation == Configuration.ORIENTATION_LANDSCAPE) {
// In landscape
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP,30F);
result.setTextSize(TypedValue.COMPLEX_UNIT_DIP,20F);
} else {
// In portrait
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP,36F);
result.setTextSize(TypedValue.COMPLEX_UNIT_DIP,26F);
}
Text size and color control function
public Void text_size_color_controller() {
display.setTextColor(Color.parseColor("#000000"));
result.setTextColor(Color.parseColor("#bdbdbd"));
if (orientation == Configuration.ORIENTATION_LANDSCAPE) {
// In landscape
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP,30F);
result.setTextSize(TypedValue.COMPLEX_UNIT_DIP,20F);
} else {
// In portrait
display.setTextSize(TypedValue.COMPLEX_UNIT_DIP,36F);
result.setTextSize(TypedValue.COMPLEX_UNIT_DIP,26F);
}
button_modulas.setEnabled(false);
return null;
}
This function control text size and color when equal button click. This function also check the device orientation for controlling the text size.
User Entry Control Function
public Void entry_controller(Boolean b) {
if(b){
button_add.setEnabled(false);
button_sub.setEnabled(false);
button_multi.setEnabled(false);
button_div.setEnabled(false);
button_equal.setEnabled(false);
}else{
button_add.setEnabled(true);
button_sub.setEnabled(true);
button_multi.setEnabled(true);
button_div.setEnabled(true);
button_equal.setEnabled(true);
}
return null;
}
This function work like this if user don’t write any number but click addition or subtraction button, this function handle this type of error. If user don’t type any number then all the operation button are disabled. Other exception is if user write like this 2+2+ and press enter then app will be crashed. This function also handle this. If user input last value is any operator then equal button automatically disabled. It also handle if user click any operator button then all the operator buttons are automatically disabled. If user need to change the operator click the back button then automatically enable all the operator buttons.
Calculation Function
public static double calculation(final String str) {
return new Object() {
int pos = -1, ch;
void nextChar() {
ch = (++pos < str.length()) ? str.charAt(pos) : -1;
}
boolean eat(int charToEat) {
while (ch == ' ') nextChar();
if (ch == charToEat) {
nextChar();
return true;
}
return false;
}
double parse() {
nextChar();
double x = parseExpression();
if (pos < str.length()) throw new RuntimeException("Unexpected: " + (char)ch);
return x;
}
// Grammar:
// expression = term | expression `+` term | expression `-` term
// term = factor | term `*` factor | term `/` factor
// factor = `+` factor | `-` factor | `(` expression `)`
// | number | functionName factor | factor `^` factor
double parseExpression() {
double x = parseTerm();
for (;;) {
if (eat('+')) x += parseTerm(); // addition
else if (eat('-')) x -= parseTerm(); // subtraction
else return x;
}
}
double parseTerm() {
double x = parseFactor();
for (;;) {
if (eat('*')) x *= parseFactor(); // multiplication
else if (eat('/')) x /= parseFactor(); // division
else return x;
}
}
double parseFactor() {
if (eat('+')) return parseFactor(); // unary plus
if (eat('-')) return -parseFactor(); // unary minus
double x;
int startPos = this.pos;
if (eat('(')) { // parentheses
x = parseExpression();
eat(')');
} else if ((ch >= '0' && ch <= '9') || ch == '.') { // numbers
while ((ch >= '0' && ch <= '9') || ch == '.') nextChar();
x = Double.parseDouble(str.substring(startPos, this.pos));
} else if (ch >= 'a' && ch <= 'z') { // functions
while (ch >= 'a' && ch <= 'z') nextChar();
String func = str.substring(startPos, this.pos);
x = parseFactor();
if (func.equals("sqrt")) x = Math.sqrt(x);
else if (func.equals("sin")) x = Math.sin(Math.toRadians(x));
else if (func.equals("cos")) x = Math.cos(Math.toRadians(x));
else if (func.equals("tan")) x = Math.tan(Math.toRadians(x));
else throw new RuntimeException("Unknown function: " + func);
} else {
throw new RuntimeException("Unexpected: " + (char)ch);
}
if (eat('^')) x = Math.pow(x, parseFactor()); // exponentiation
return x;
}
}.parse();
}
When user click equal button then equal button onClickListener function get the all user input as string then pass it int this calculation function. This function phrase the string and calculate the value and return result as decimal data type.
Leave a Comment
(0 Comments)