ListView in Android Studio Kotlin
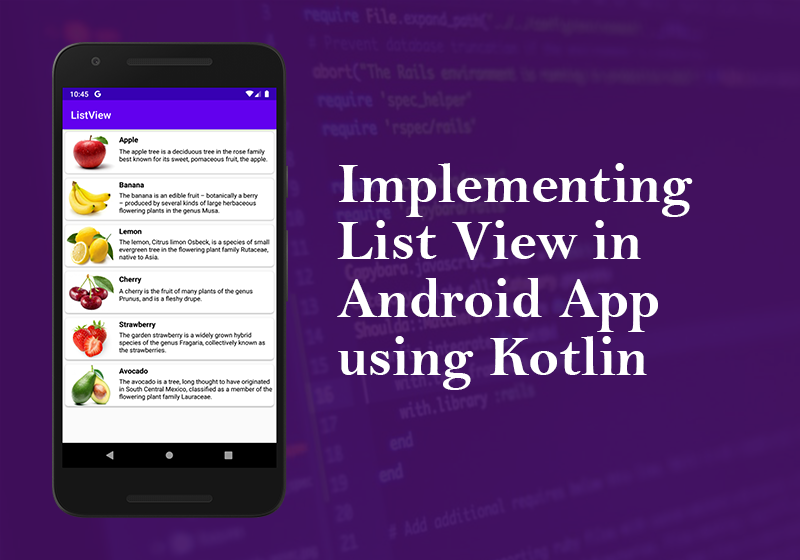
Using a Custom Adapter
This time we need to change your activity_list_item.xml file. Just copy the bellow code and paste
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<androidx.cardview.widget.CardView
android:layout_height="match_parent"
android:layout_width="match_parent"
android:layout_margin="5dp"
app:cardCornerRadius="6dp"
app:cardElevation="2dp" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="80dp"
android:orientation="horizontal"
android:weightSum="4">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1">
<ImageView
android:id="@+id/image"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitXY"
android:src="@mipmap/ic_launcher" />
</LinearLayout>
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_weight="3"
android:layout_marginLeft="5dp"
android:weightSum="3">
<TextView
android:id="@+id/item"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="left|center"
android:text="Fruit name"
android:textStyle="bold"
android:textColor="#000000" />
<TextView
android:id="@+id/subitem"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="2"
android:layout_gravity="center"
android:text="fruit list"
android:textSize="12dp"
android:textColor="#000000" />
</LinearLayout>
</LinearLayout>
</androidx.cardview.widget.CardView>
</LinearLayout>
First we create a java class name CustomAdapter. Inside CustomAdapter.kt file copy and paste the bellow code without changing package name
package com.andrious.listview
/**
* Created by MD.ISRAFIL MAHMUD on 06/25/2020.
*/
import android.content.Context
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.BaseAdapter
import android.widget.ImageView
import android.widget.TextView
class CustomAdapter(private val context: Context?,
private val Item: Array<String>,
private val SubItem: Array<String>,
private val flags: IntArray) : BaseAdapter() {
private val inflater: LayoutInflater = this.context!!.getSystemService(Context.LAYOUT_INFLATER_SERVICE) as LayoutInflater
override fun getCount(): Int { return Item.size }
override fun getItem(position: Int): Int { return position }
override fun getItemId(position: Int): Long { return position.toLong() }
override fun getView(position: Int, convertView: View?, parent: ViewGroup): View {
val rowView = inflater.inflate(R.layout.activity_list_item, parent, false)
rowView.findViewById<TextView>(R.id.item).text = Item.get(position)
rowView.findViewById<TextView>(R.id.subitem).text = SubItem.get(position)
val image: ImageView = rowView.findViewById(R.id.image) as ImageView
flags?.get(position)?.let { image.setImageResource(it) }
rowView.tag = position
return rowView
}
}
Few change into our MainActivity.kt file. Create two string type array one for Item name and one for Item description. One int type array for holding drawable food image name. Look like this
var Item = arrayOf("Apple", "Banana", "Lemon", "Cherry", "Strawberry", "Avocado")
var SubItem = arrayOf("The apple tree is a deciduous tree in the rose family best known for its sweet, pomaceous fruit, the apple.",
"The banana is an edible fruit – botanically a berry – produced by several kinds of large herbaceous flowering plants in the genus Musa.",
"The lemon, Citrus limon Osbeck, is a species of small evergreen tree in the flowering plant family Rutaceae, native to Asia.",
"A cherry is the fruit of many plants of the genus Prunus, and is a fleshy drupe.",
"The garden strawberry is a widely grown hybrid species of the genus Fragaria, collectively known as the strawberries.",
"The avocado is a tree, long thought to have originated in South Central Mexico, classified as a member of the flowering plant family Lauraceae.")
Now we need some pictuer into our Item for creating int type array for holding drawable image name for each Item.
Download this images and paste into your drawable folder. You can also be able to download images from google or any other site for your satisfaction.
Now we declare int array for holding drawable image name for each Item.
var flags = intArrayOf(
R.drawable.apple,
R.drawable.banana,
R.drawable.lemon,
R.drawable.cherry,
R.drawable.strawberrie,
R.drawable.avocado
)
Now, we just need to connect this adapter to a ListView to be populated:
val customAdapter = CustomAdapter(applicationContext, Item, SubItem, flags)
val simpleList = findViewById(R.id.ListView) as ListView
simpleList.adapter = customAdapter
simpleList.onItemClickListener = OnItemClickListener { arg0, v, position, id ->
// TODO Auto-generated method stub
Toast.makeText(applicationContext, Item[position], Toast.LENGTH_SHORT).show()
}
Our MainActivity.kt file look this
package com.andrious.listview
import android.os.Bundle
import android.widget.AdapterView.OnItemClickListener
import android.widget.ListView
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
var Item = arrayOf("Apple", "Banana", "Lemon", "Cherry", "Strawberry", "Avocado")
var SubItem = arrayOf("The apple tree is a deciduous tree in the rose family best known for its sweet, pomaceous fruit, the apple.",
"The banana is an edible fruit – botanically a berry – produced by several kinds of large herbaceous flowering plants in the genus Musa.",
"The lemon, Citrus limon Osbeck, is a species of small evergreen tree in the flowering plant family Rutaceae, native to Asia.",
"A cherry is the fruit of many plants of the genus Prunus, and is a fleshy drupe.",
"The garden strawberry is a widely grown hybrid species of the genus Fragaria, collectively known as the strawberries.",
"The avocado is a tree, long thought to have originated in South Central Mexico, classified as a member of the flowering plant family Lauraceae.")
var flags = intArrayOf(
R.drawable.apple,
R.drawable.banana,
R.drawable.lemon,
R.drawable.cherry,
R.drawable.strawberrie,
R.drawable.avocado
)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val customAdapter = CustomAdapter(applicationContext, Item, SubItem, flags)
val simpleList = findViewById(R.id.ListView) as ListView
simpleList.adapter = customAdapter
simpleList.onItemClickListener = OnItemClickListener { arg0, v, position, id ->
// TODO Auto-generated method stub
Toast.makeText(applicationContext, Item[position], Toast.LENGTH_SHORT).show()
}
}
}
When you run this your application will look like this
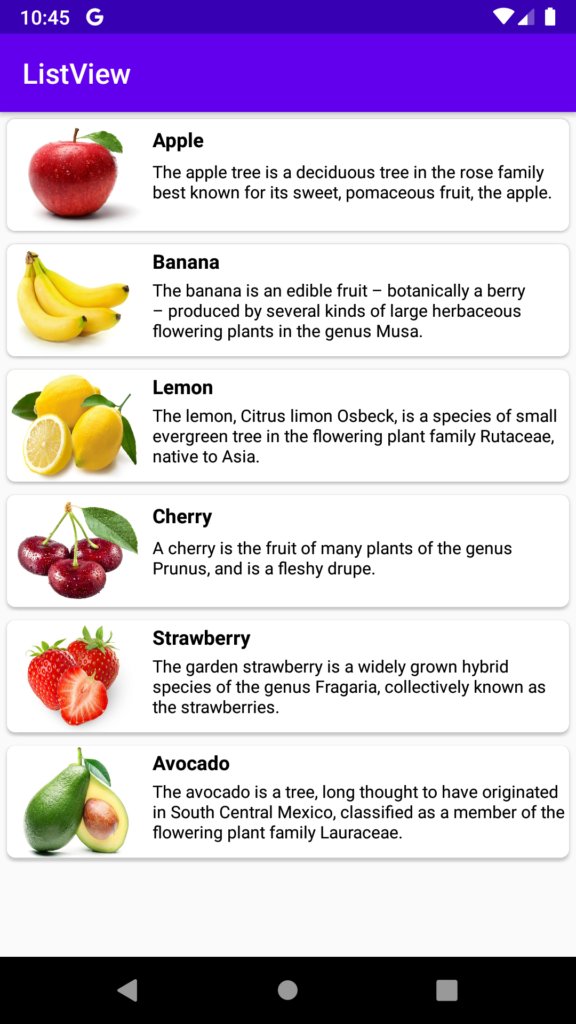
Leave a Comment
(0 Comments)